지난 1편에 이어서 안드로이드 컴포즈에 대해 알아보겠다.
이번에 알아볼 내용은 기본 중의 기본인 Text를 알아보겠다.
# Android Compose Text()
컴포즈의 Text는 Xml의 TextView라고 보면 된다.
Text = TextView
Text()를 자세히 살펴보면 아래와 같은 많은 속성들이 있다.
text: AnnotatedString,
modifier: Modifier = Modifier,
color: Color = Color.Unspecified,
fontSize: TextUnit = TextUnit.Unspecified,
fontStyle: FontStyle? = null,
fontWeight: FontWeight? = null,
fontFamily: FontFamily? = null,
letterSpacing: TextUnit = TextUnit.Unspecified,
textDecoration: TextDecoration? = null,
textAlign: TextAlign? = null,
lineHeight: TextUnit = TextUnit.Unspecified,
overflow: TextOverflow = TextOverflow.Clip,
softWrap: Boolean = true,
maxLines: Int = Int.MAX_VALUE,
inlineContent: Map<String, InlineTextContent> = mapOf(),
onTextLayout: (TextLayoutResult) -> Unit = {},
style: TextStyle = LocalTextStyle.current
이중 대표적인 속성들(color, fontSize, fontStyle, fontWeight, textAligen, maxLines, overflow)이 무슨 역할을 하는지 살펴보겠다.
# text
- 표시 할 문자를 적어주는 곳이다.
- text안에 문자열 리소스를 사용하고 싶으면 stringResource(R.string.hello_world)처럼 적어 주면 된다.
※ 예시 코드 + 이미지
- MainActivity.kt
package com.example.blogcompose
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Arrangement.Center
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material.MaterialTheme
import androidx.compose.material.Surface
import androidx.compose.material.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.stringResource
import com.example.blogcompose.ui.theme.BlogComposeTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
BlogComposeTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colors.background
) {
TestText()
}
}
}
}
}
// Text를 보여주는 @Composable 생성
@Composable
fun TestText() {
Column(
verticalArrangement = Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Text(text = "안녕하세요")
Text(text = stringResource(R.string.comment_test_text))
}
}
- strings.xml
<resources>
<string name="app_name">blogCompose</string>
<string name="comment_test_text">문자열 리소스 사용</string>
</resources>
우선 Composable안에 있는 Column함수는 다음에 포스팅하겠다.
지금은 Text 함수를 집중적으로 보길 바란다.
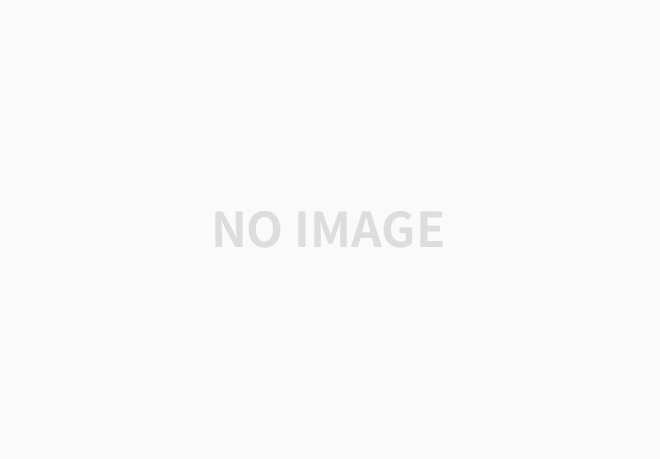
# modifer
- Text함수만의 속성이 아니고 Composable 거의 모든 곳에 사용할 수 있다
- 크기, 레이아웃, 동작 및 모양 변경 / 접근성 라벨과 같은 정보 추가 / 사용자 입력 처리,
UI 요소를 클릭 가능, 스크롤 가능, 드래그 가능 또는 확대/축소 가능하게 만드는 것과 같은 높은 수준의 상호작용 추가를 할 수 있다.
예시로는 많은 기능들이 있는데 그중 대표적인 것(width, height, padding, background)만 해보겠다.
※ 예시 코드 + 이미지
// Text를 보여주는 @Composable 생성
@Composable
fun TestText() {
Column(
verticalArrangement = Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Text(
text = "안녕하세요",
modifier = Modifier
.background(Color.Blue) // 배경을 ()안에 있는걸로 교체
.fillMaxWidth() // 가로로 꽉차게 xml = match parent
.padding(bottom = 25.dp) // bottom 간격을 25.dp 만큼 띄운다
)
Text(
text = stringResource(R.string.comment_test_text),
modifier = Modifier
.background(Color.Green) // 배경을 ()안에 있는걸로 교체
.width(300.dp) // 가로 크기를 300dp로 설정
.height(50.dp) // 세로 크기를 50dp로 설정
)
}
}
modifier안에 있는 속성은 주석으로 적어놨으니 참고하길 바란다.
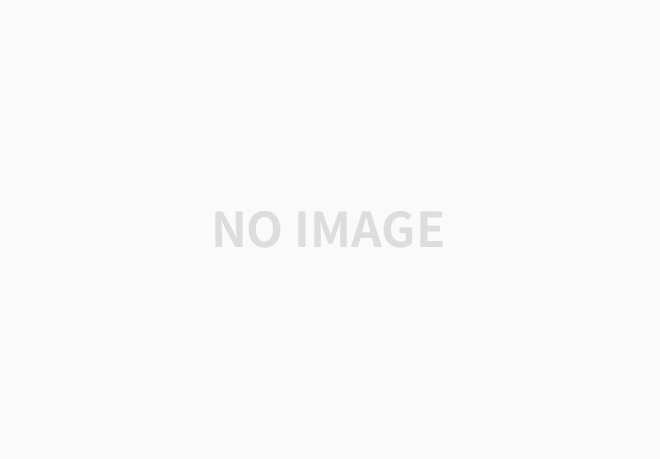
# color
- 텍스트의 색상을 변경하는 함수이다.
※ 예시 코드 + 이미지
// Text를 보여주는 @Composable 생성
@Composable
fun TestText() {
Column(
verticalArrangement = Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Text(
text = "안녕하세요",
modifier = Modifier.padding(bottom = 25.dp), // bottom 간격을 25.dp 만큼 띄운다
color = Color.Blue // text 색상을 파란색으로 설정
)
Text(
text = stringResource(R.string.comment_test_text),
color = Color.Red // text 색상을 빨간색으로 설정
)
}
}
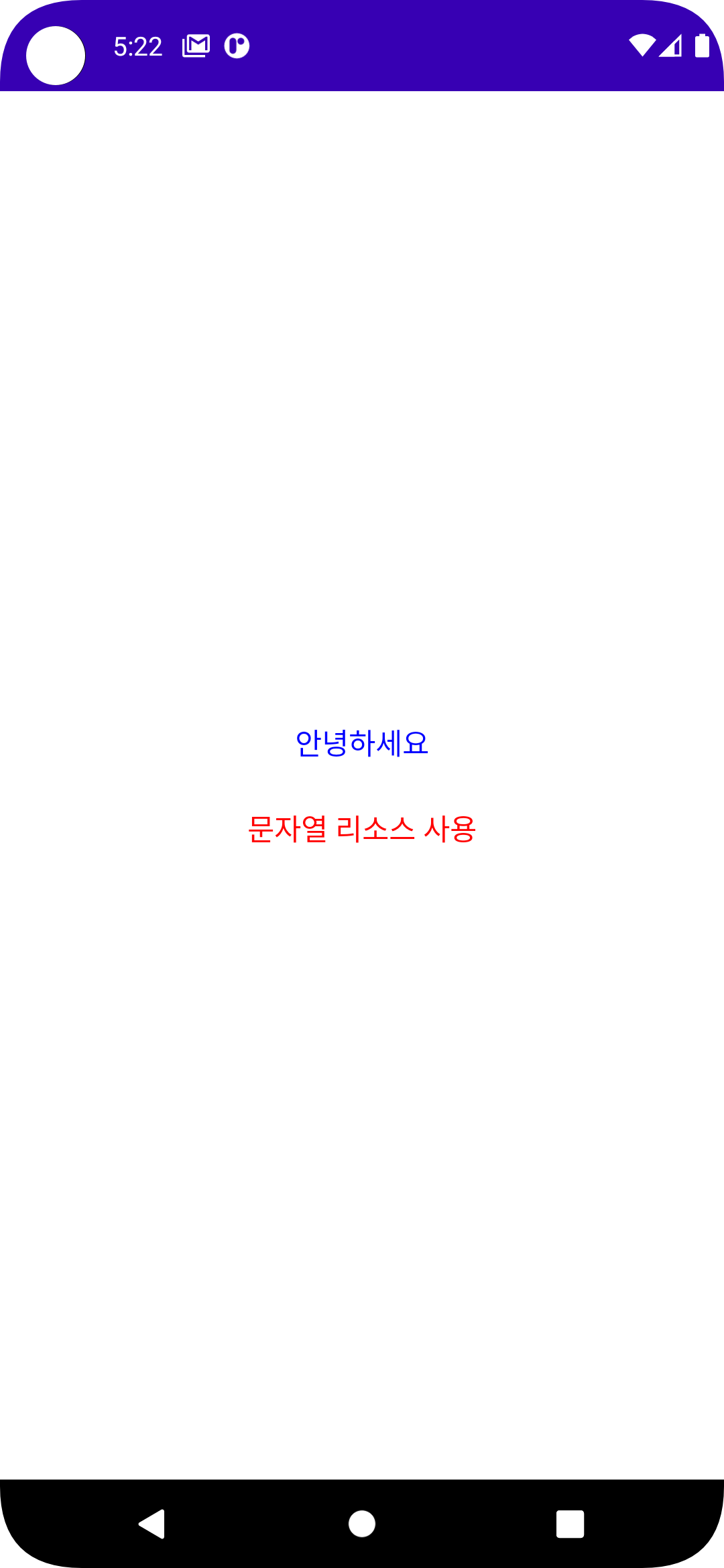
# fontSize
- 텍스트의 크기를 바꾸는 속성이다.
※ 예시 코드 + 이미지
Text(
text = "안녕하세요",
modifier = Modifier.padding(bottom = 25.dp),
color = Color.Blue, // text 색상을 파란색으로 설정
fontSize = 60.sp // text 크기를 60sp로 설정
)
Text(
text = stringResource(R.string.comment_test_text),
color = Color.Red, // text 색상을 빨간색으로 설정
fontSize = 20.sp // text 크기를 20sp로 설정
)
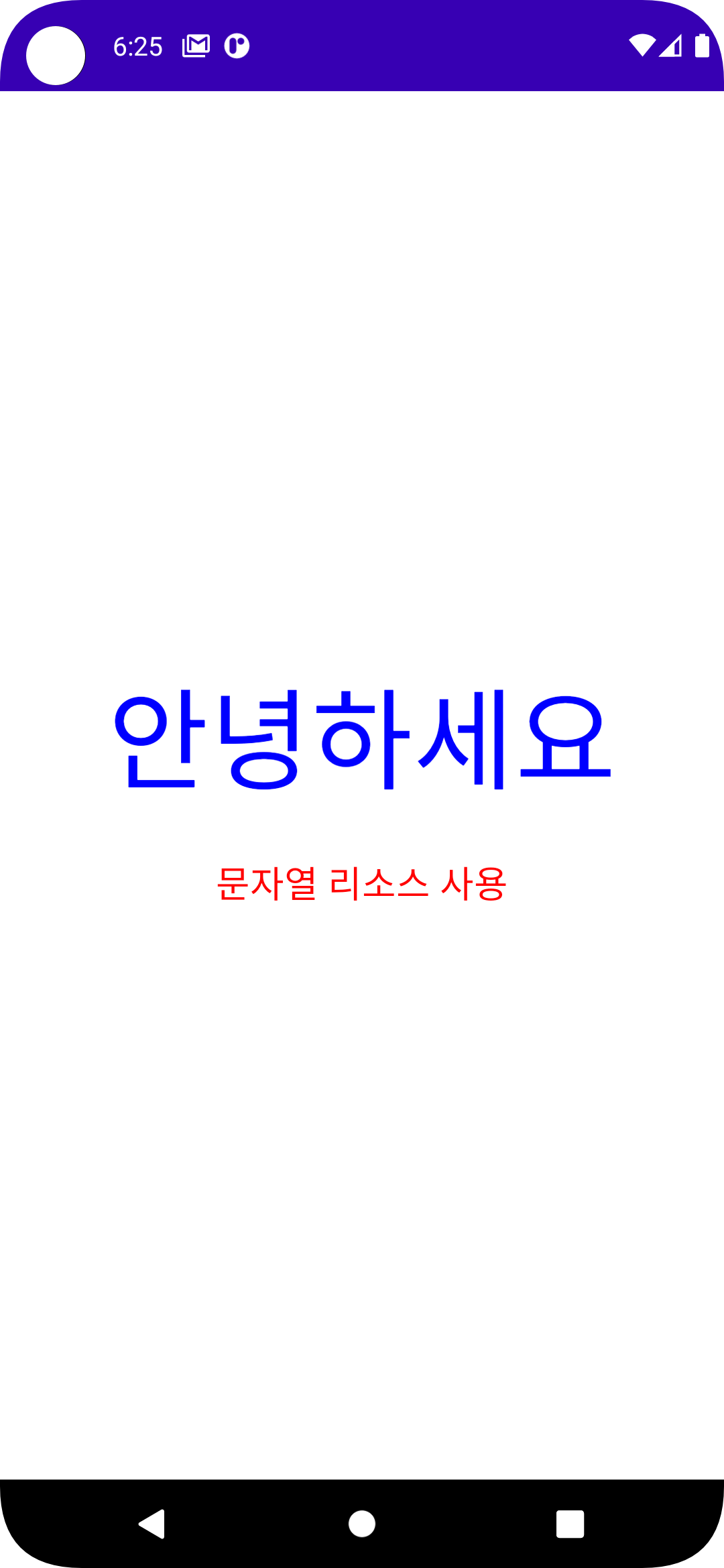
# fontStyle , fontWeight
- fontStyle : 텍스트를 기울임꼴로 표시할 수 있다.
- fontWeight : 텍스트를 굵게 표시 할 수 있다. (Bold, Black, ExtraBold, ExtraLight, Light, Medium, SemiBold, Thin)등 많은 표시 옵션이 있다.
※ 예시 코드 + 이미지
Text(
text = "안녕하세요",
fontStyle = FontStyle.Italic // fontStyle로 기울임꼴 표시
)
Text(
text = stringResource(R.string.comment_test_text),
fontWeight = FontWeight.Bold // text를 굵게 설정
)
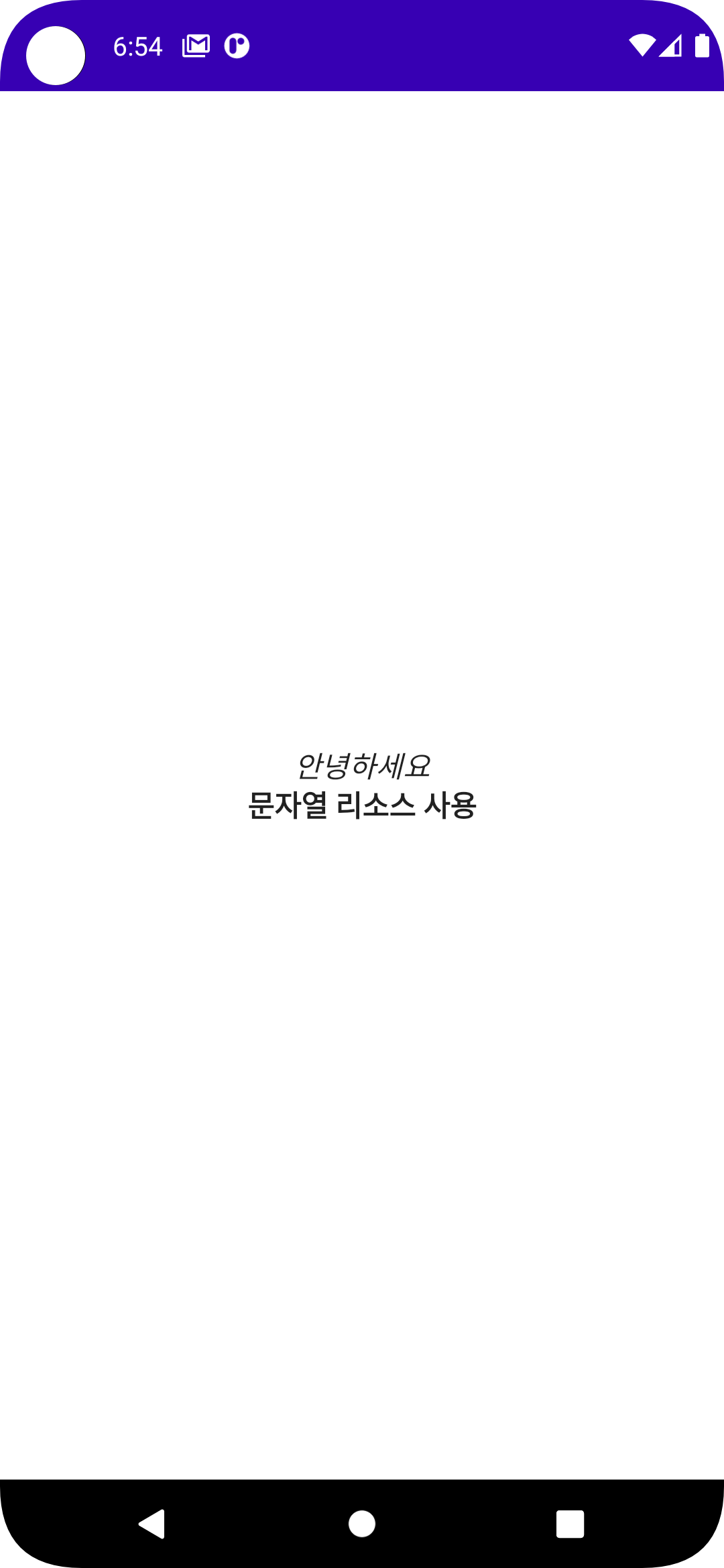
# textAligen
- 텍스트의 정렬을 설정 할 수 있다.
- 텍스트의 정렬을 하기 위해서는 정렬을 하기 위한 공간이 있어야 한다.
※ 예시 코드 + 이미지
Text(
text = "왼쪽 정렬",
Modifier.fillMaxWidth(), // text 정렬을 위한 가로를 꽉차게 설정
textAlign = TextAlign.Start // 왼쪽 정렬
)
Text(
text = "가운데 정렬",
Modifier.fillMaxWidth(),// text 정렬을 위한 가로를 꽉차게 설정
textAlign = TextAlign.Center // 가운데 정렬
)
Text(
text = "오른쪽 정렬",
Modifier.fillMaxWidth(),// text 정렬을 위한 가로를 꽉차게 설정
textAlign = TextAlign.End // 오른쪽 정렬
)
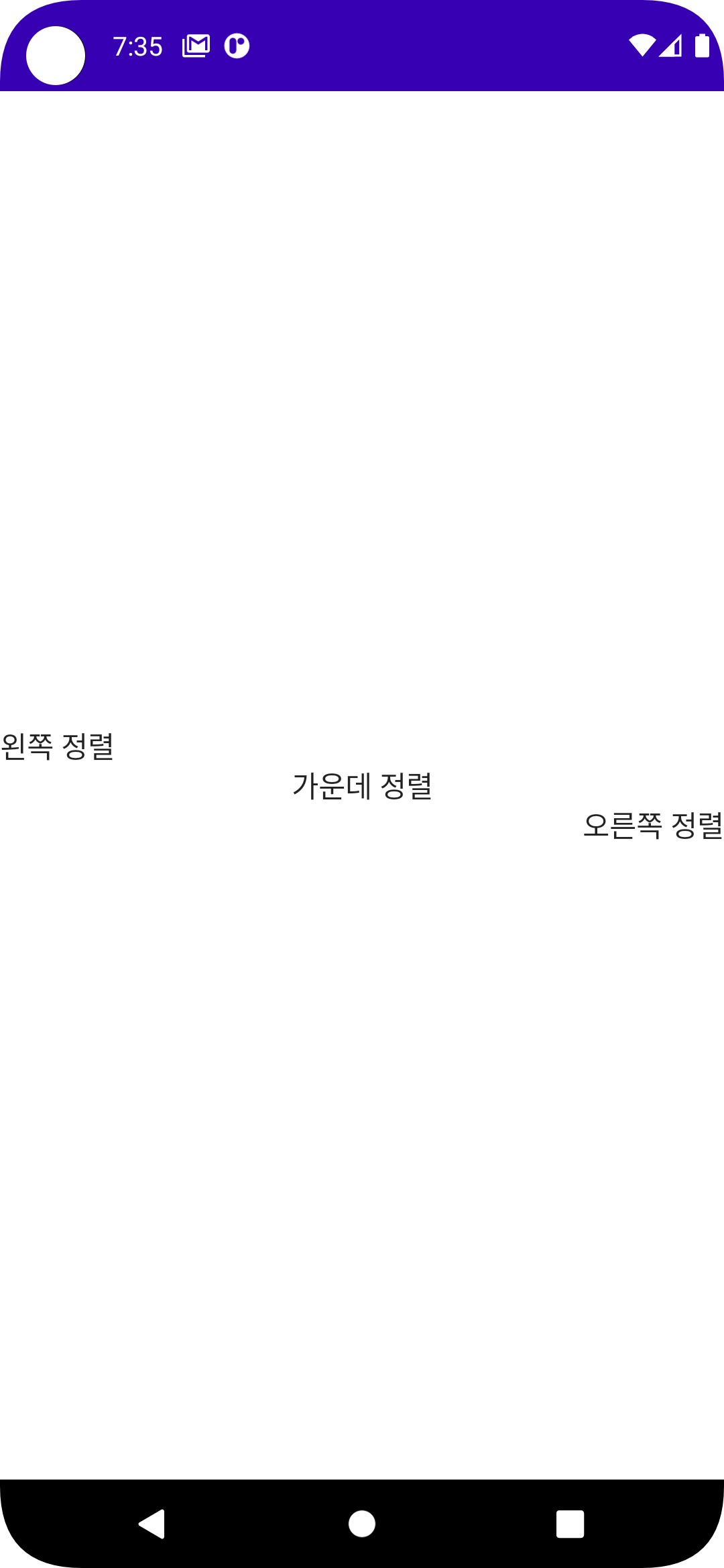
# maxLines, overflow
- maxLines : 표시되는 줄 수를 제한할 수 있다.
- overflow : 텍스트가 설정한 줄 수를 넘어가게 되면 표시할 옵션을 설정할 수 있다.
※ 예시 코드 + 이미지
Text(
text = "테스트1".repeat(100),
maxLines = 1, // 최대 1줄
overflow = TextOverflow.Clip, // TextOverflow Clip로 설정
)
Text(
text = "테스트2".repeat(100),
maxLines = 2, // 최대 2줄
overflow = TextOverflow.Ellipsis, // TextOverflow Ellipsis로 설정
)
Text(
text = "테스트3".repeat(100),
maxLines = 3, // 최대 3줄
overflow = TextOverflow.Visible, // TextOverflow Visible로 설정
)
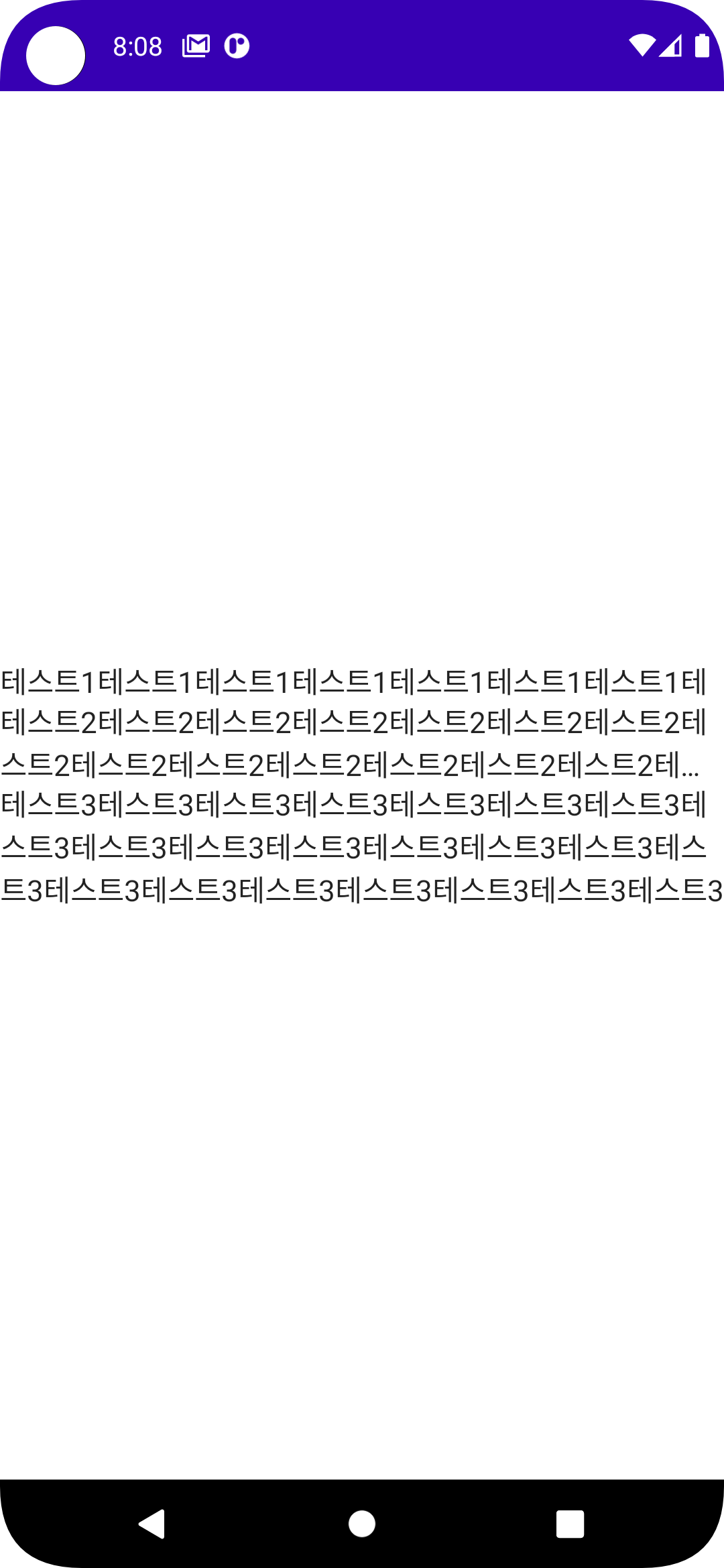
정리를 하자면 아래 표와 같다
속성 | 내용 |
text | 표시 할 문자를 적어주는 곳이다. |
modifer | Text함수만의 속성이 아니고 Composable 거의 모든 곳에 사용할 수 있다 크기, 레이아웃, 동작 및 모양 변경 / 접근성 라벨과 같은 정보 추가 / 사용자 입력 처리, UI 요소를 클릭 가능, 스크롤 가능, 드래그 가능 또는 확대/축소 가능하게 만드는 것과 같은 높은 수준의 상호작용 추가를 할 수 있다. |
color | 텍스트의 색상을 변경하는 함수이다. |
fontSize | 텍스트의 크기를 변경하는 함수이다. |
fontStyle | 텍스트를 기울임꼴로 표시 할 수 있다. |
fontWeight | 텍스트를 굵게 표시 할 수 있다. (Bold,Black,ExtraBold,ExtraLight,Light,Medium,SemiBold,Thin)등 많은 표시 옵션이 있다. |
textAligen | 텍스트의 정렬을 설정 할 수 있다. 텍스트의 정렬을 하기 위해서는 정렬을 하기위한 공간이 있어야한다. |
maxLines | 표시되는 줄 수를 제한 할 수 있다. |
overflow | 텍스트가 설정한 줄 수를 넘어가게 되면 표시 할 옵션을 설정할 수 있다. |
'안드로이드 공부 노트 > Compose(컴포즈)' 카테고리의 다른 글
[Android-Compose] 안드로이드 컴포즈 Text HyperLink (텍스트 하이퍼링크) 적용하기 (0) | 2023.01.15 |
---|---|
[Android-Compose] 안드로이드 컴포즈 5편 예제를 통한 Scaffold 알아보기! - topBar (0) | 2022.12.18 |
[Android-Compose] 안드로이드 컴포즈 4편 Lazy lists - LazyColumn, LazyRow (0) | 2022.11.06 |
[Android-Compose] 안드로이드 컴포즈 3편 - Row,Column (0) | 2022.09.04 |
[Android-Compose] 안드로이드 컴포즈 1편(컴포즈란?) (0) | 2022.07.24 |